Index
- Initial Setup
1.1 Clone Project
- Running Tests
4.1 Running tests by Tag
4.2 Running more than one Tag
1. Initial Setup
1.1 Clone Project
We are going to continue from a previous automation project “Gherkin with Python and Pytest-BDD”.
Make sure you clone the right branch so we can start: “tutorial/gherkin-pytest-bdd”.
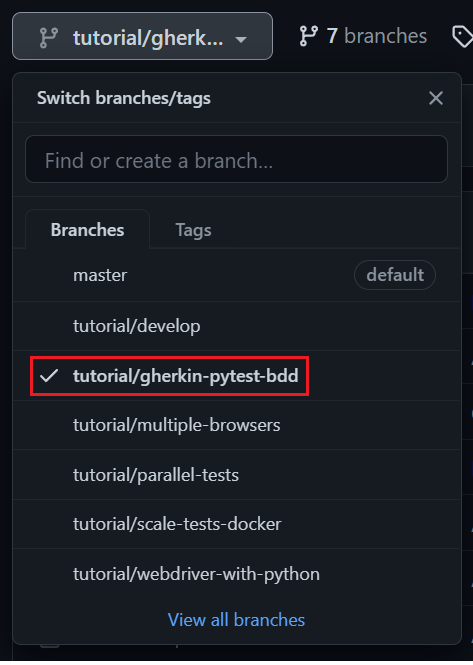
After cloning it, check in the VSCode if you cloned the right branch.
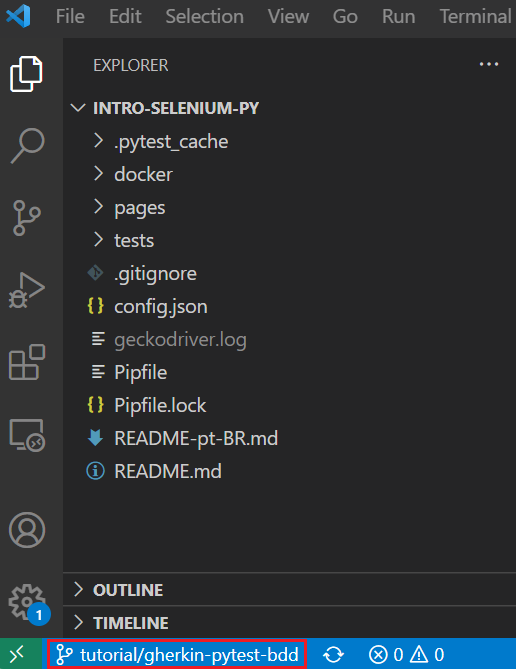
2. Filtering Tests with Tags
Tags should be applied in the gherkin files, they can be applied to:
- Features.
- Scenarios.
- Scenario Outlines.
2.1 Example of Tag Implementation
A feature or scenario can have an unlimited number of tags.
@web @basic-search
Feature: DuckDuckGo Web Browsing
The same tags can be used by more than one feature or scenario as well.
@web
Feature: DuckDuckGo Web Browsing
@web
Scenario: Basic DuckDuckGo Result Title
Tags added to features affect the scenarios inside the features.
@web
Feature: DuckDuckGo Web Browsing
As a web surfer,
I want to find information online,
So I can learn new things and get tasks done.
Background:
Given the DuckDuckGo home page is displayed
Scenario: Basic DuckDuckGo Result Title
When the user searches for "panda"
Then results title contains "panda"
3. Implementation
“web.feature”:
@web
Feature: DuckDuckGo Web Browsing
As a web surfer,
I want to find information online,
So I can learn new things and get tasks done.
Background:
Given the DuckDuckGo home page is displayed
@basic-search
Scenario: Basic DuckDuckGo Result Title
When the user searches for "panda"
Then results title contains "panda"
@scenario-outline
Scenario Outline: Basic DuckDuckGo Search
When the user searches for <name>
Then results are shown for <found_animal>
Examples: Animals
| name | found_animal |
| panda | panda |
| python | python |
| polar bear | polar bear |
@basic-search @independence-search
Scenario: Lengthy DuckDuckGo Search
When the user searches for the phrase:
"""
When in the Course of human events, it becomes necessary for one people
to dissolve the political bands which have connected them with another,
and to assume among the powers of the earth, the separate and equal
station to which the Laws of Nature and of Nature's God entitle them,
a decent respect to the opinions of mankind requires that they should
declare the causes which impel them to the separation.
"""
Then one of the results contains "Declaration of Independence"
4. Running Tests
4.1 Running tests by Tag
Open the “Command Prompt” in your project root and write the command below.
Remember to remove the at symbol (@) that came before the tag name, also remember to put the tag name between double quotes.
pipenv run python -m pytest -k "web"
After running it, maybe the test can throw a warning.
PytestUnknownMarkWarning: Unknown pytest.mark.xxx - is this a typo?
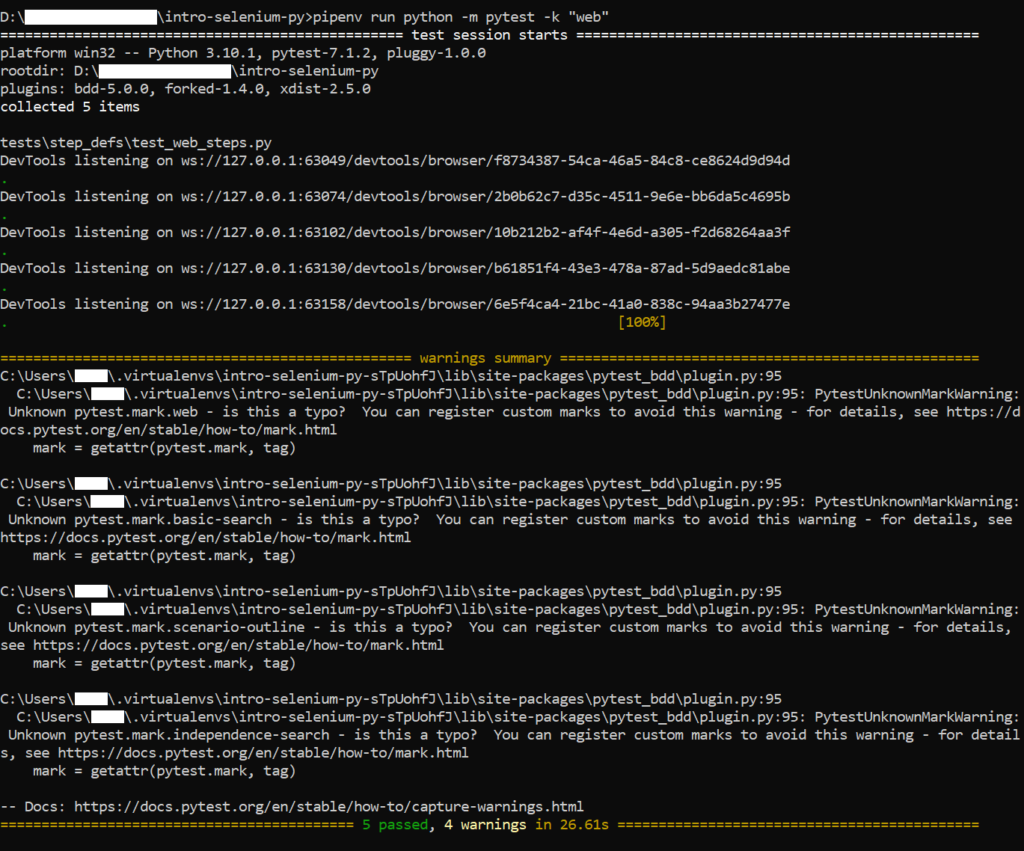
If the warning above is shown, create the file “pytest.ini” inside the project root and write your project tags in it.
“pytest.ini”:
[pytest]
markers =
web: marks tests as web tests
basic-search
scenario-outline
independence-search
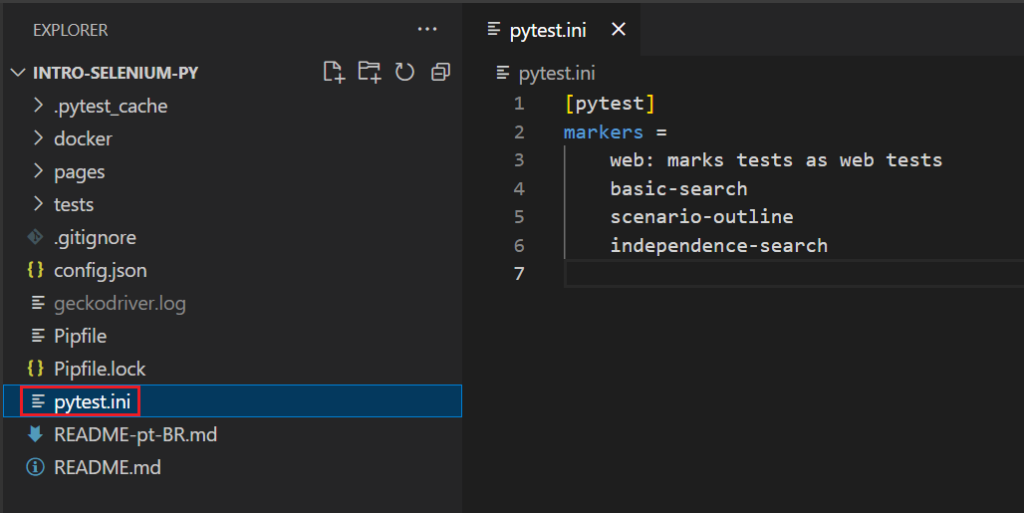
Run the test again and all the scenarios under the @web tag will be executed.
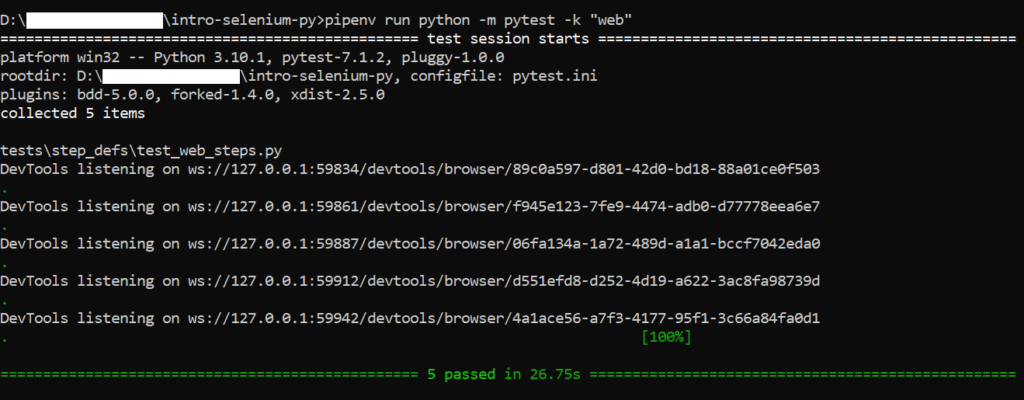
4.2 Running more than one Tag
You can use python logic operators (and, or, not) to specify which tag should be called.
- And.
Run all the tests that have both the tags “basic-search” and “independence-search”.
pipenv run python -m pytest -k "basic-search and independence-search"
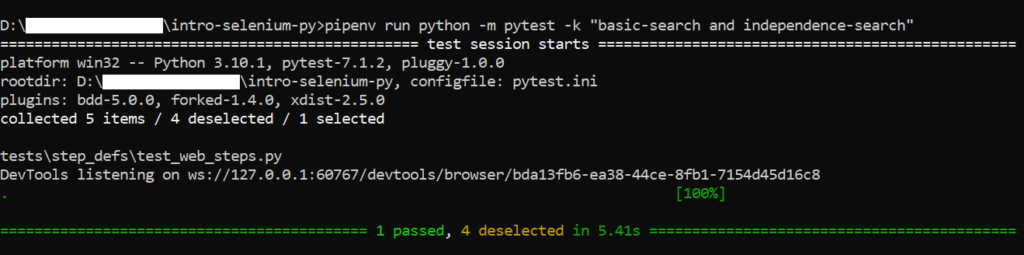
- Or.
Run all tests that have one tag or another: “scenario-outline” or “independence-search”.
pipenv run python -m pytest -k "scenario-outline or independence-search"
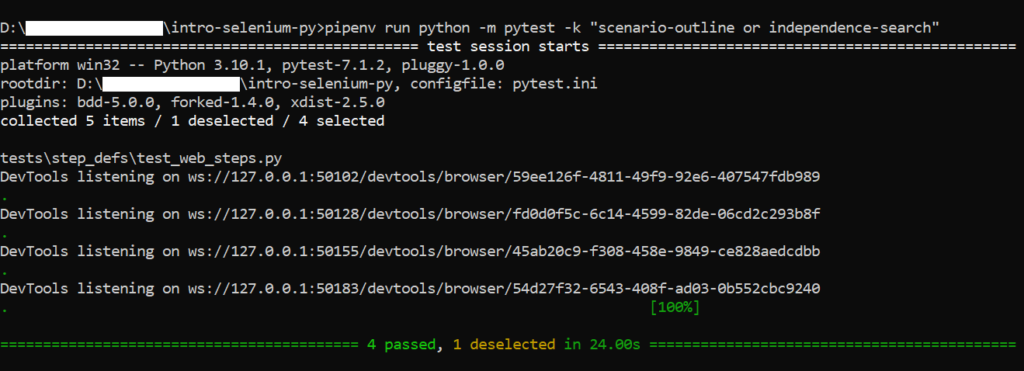
- Not.
Run tests that have the tag “basic-search” and do not run tests tagged with “scenario-outline”.
pipenv run python -m pytest -k "basic-search and not scenario-outline"
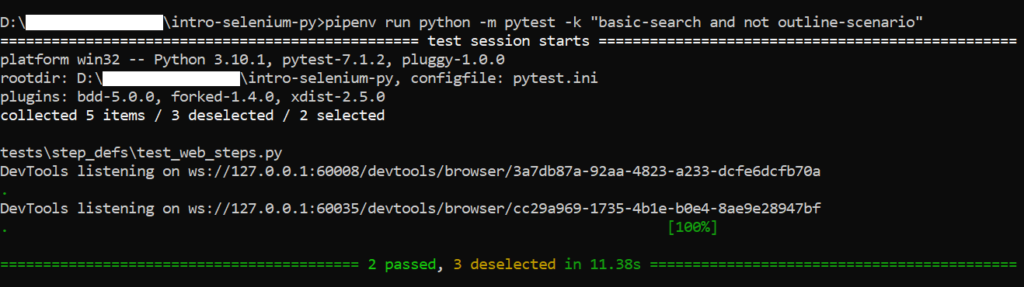
5. Repository
I created a new branch called “tutorial/gherkin-tags” for this tutorial, click the link below to see it:
https://github.com/LuizGustavoR/intro-selenium-py/tree/tutorial/gherkin-tags
The end.
6. Thanks
- This guide was created based on the course “Behavior Driven Python with pytest-bdd” by Andrew Knight.
- Course available at “Testing Automation University”.